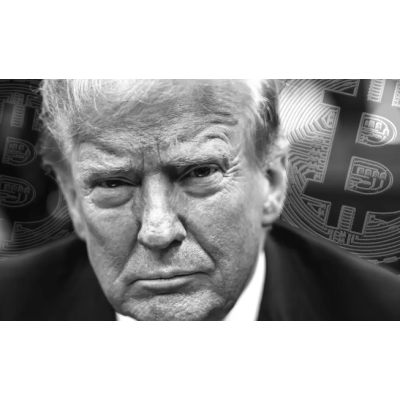
TWS (Trader Workstation) by Interactive Brokers is a powerful platform that provides access to a wide range of financial instruments and markets. It offers extensive capabilities for algorithmic trading, including real-time market data, order execution, and account management functionalities.
Before diving into coding, ensure you have Python installed on your system. Additionally, you’ll need to install the necessary libraries such as ibapi
, ccxt
, pandas
, and binance
. These libraries facilitate communication with the TWS API, fetching historical data, and handling account balances.
import ibapi
import queue
from ibapi.client import *
from ibapi.wrapper import *
import threading
from math import *
import pandas as pd
import ccxt
import numpy as np
import time
from binance.client import Client
from ibapi.contract import Contract
from ibapi.order import Order
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
The first step in building our trading bot is establishing a connection with the TWS API. We create a custom class inheriting from EWrapper
and EClient
, which allows us to handle server responses and send requests to the TWS server.
class IBapi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
We define functions to create order and contract objects, specifying details such as symbol, security type, exchange, currency, quantity, and price. These objects encapsulate the parameters required to place orders with TWS.
def make_contract(symbol, sec_type, exch, prim_exch, curr):
Contract.m_symbol = symbol
Contract.m_secType = sec_type
Contract.m_exchange = exch
Contract.m_primaryExch = prim_exch
Contract.m_currency = curr
return Contract
def make_order(action, quantity, price=None):
if price is not None:
order = Order()
order.m_orderType = 'LMT'
order.m_totalQuantity = quantity
order.m_action = action
order.m_lmtPrice = price
else:
order = Order()
order.m_orderType = 'MKT'
order.m_totalQuantity = quantity
order.m_action = action
return order
To make informed trading decisions, historical data is essential. We utilize the ccxt
library to fetch historical price data for the desired financial instrument, such as Bitcoin (BTC) against the US Dollar (USD), from the Binance exchange.
client = Client()
symbol = "BTCUSDT"
klines = client.get_historical_klines(symbol, Client.KLINE_INTERVAL_1DAY, "80 days ago UTC")
df= pd.DataFrame(klines, columns = ['timestamp', 'open', 'high', 'low', 'close', 'volume','close_time', 'quote_av', 'trades', 'tb_base_av', 'tb_quote_av', 'ignore' ])
# (Continued below...)
In this example, we demonstrate a simple trading strategy based on pivot points. We calculate pivot points and support/resistance levels from historical price data and define buy and sell conditions based on these levels.
# (Continuation from above...)
# Implementing trading strategy
df['close1'] = df['close'].shift(1)
df['open1'] = df['open'].shift(1)
# (Continued below...)
Account management is crucial in algorithmic trading. We fetch account balances using the TWS API and ensure we have sufficient funds (USD) or assets (BTC) available for trading.
ib_api = IBapi()
ib_api.connect("127.0.0.1", 7497, clientId=1)
# (Continued below...)
Based on the predefined trading strategy and account balances, we implement logic to place buy and sell orders with TWS. We specify order types (market or limit) and adjust stop-loss levels dynamically to manage risk.
# (Continuation from above...)
# Placing buy and sell orders
if x == 1:
if Portfolio > 10:
conn = Connection.create(port=7496, clientId=999)
conn.connect()
oid = 1
cont = make_contract('BTC', 'STK', 'SMART', 'SMART', 'USD')
offer = make_order('BUY', 1, 200)
conn.placeOrder(oid, cont, offer)
conn.disconnect()
print("OPEN A LONG", actualPrice)
sl = df.iloc[-2]['close'] * 0.97
time.sleep(5)
else:
pass
# (Continued below...)
To ensure robustness, we implement error handling mechanisms to catch and handle exceptions gracefully. Additionally, we incorporate a retry mechanism to retry failed operations after a short delay.
try:
# (Your code block here...)
except Exception as e:
print(f"An error occurred: {str(e)}")
# Add appropriate error handling or logging here
time.sleep(300) # Retry after 5 minutes
Building a trading bot with TWS Interactive Brokers empowers traders to automate their strategies and execute trades with precision and speed. By following the steps outlined in this article and leveraging Python’s capabilities, you can create a powerful trading bot tailored to your specific requirements and preferences.