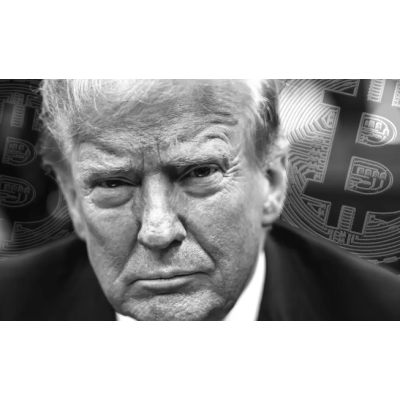
requests
, websocket-client
, and numpy
. You can install them using:pip install requests websocket-client numpy
import requests
import websocket
import json
Use WebSocket to connect to Binance’s API for real-time data. You’ll typically listen to the wss://stream.binance.com:9443/ws
endpoint.
def on_message(ws, message):
print(json.loads(message))
def on_error(ws, error):
print(error)
def on_close(ws):
print("### closed ###")
def on_open(ws):
print("### connected ###")
if __name__ == "__main__":
websocket.enableTrace(True)
ws = websocket.WebSocketApp("wss://stream.binance.com:9443/ws/btcusdt@trade",
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
ws.run_forever()
Use your API keys to authenticate with Binance’s API. You’ll include these keys in the request headers when making API calls.
api_key = 'your_api_key'
api_secret = 'your_api_secret'
headers = {
'X-MBX-APIKEY': api_key
}
Develop your trading strategies based on market data received from Binance. This could involve technical indicators, statistical models, or machine learning algorithms.
# Example: Simple Moving Average (SMA) crossover strategy
def sma_crossover_strategy(data, short_window=50, long_window=200):
signals = pd.DataFrame(index=data.index)
signals['signal'] = 0.0
# Calculate short and long SMA
signals['short_mavg'] = data['Close'].rolling(window=short_window, min_periods=1, center=False).mean()
signals['long_mavg'] = data['Close'].rolling(window=long_window, min_periods=1, center=False).mean()
# Generate signals
signals['signal'][short_window:] = np.where(signals['short_mavg'][short_window:] > signals['long_mavg'][short_window:], 1.0, 0.0)
# Generate trading orders
signals['positions'] = signals['signal'].diff()
return signals
Based on your trading logic, place buy/sell orders through Binance’s API using the POST /api/v3/order
endpoint.
def place_order(symbol, side, quantity, order_type='MARKET'):
url = 'https://api.binance.com/api/v3/order'
params = {
'symbol': symbol,
'side': side,
'type': order_type,
'quantity': quantity,
'timestamp': int(time.time() * 1000)
}
# Add signature
query_string = '&'.join([f"{k}={v}" for k, v in params.items()])
signature = hmac.new(api_secret.encode(), query_string.encode(), hashlib.sha256).hexdigest()
params['signature'] = signature
response = requests.post(url, headers=headers, params=params)
return response.json()
Consider factors such as position sizing, stop-loss orders, and leverage to manage risk effectively.
# Example: Position sizing based on portfolio size and risk percentage
def calculate_position_size(portfolio_size, risk_percentage, entry_price, stop_loss_price):
risk_amount = portfolio_size * (risk_percentage / 100)
position_size = risk_amount / (entry_price - stop_loss_price)
return position_size
Continuously monitor the execution of your trading bot to ensure it behaves as expected and reacts appropriately to market conditions.
# Example: Monitor order execution
def monitor_execution(order_id):
url = f'https://api.binance.com/api/v3/order'
params = {
'symbol': symbol,
'orderId': order_id,
'timestamp': int(time.time() * 1000)
}
response = requests.get(url, headers=headers, params=params)
return response.json()
This guide provides a foundational framework for building a high-frequency trading bot using Python and Binance’s API. However, keep in mind that high-frequency trading involves significant complexity, and successful implementation requires thorough testing, risk management, and continuous optimization. Additionally, always ensure compliance with relevant regulations and exchange policies.