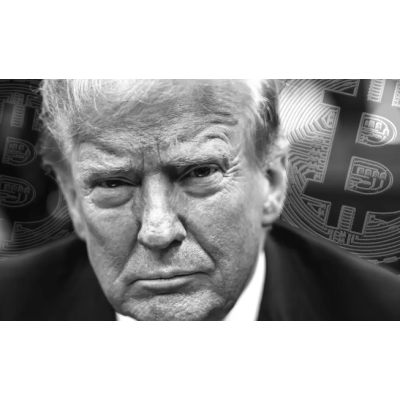
The Average Directional Movement indicator is a popular technical indicator that helps traders understand the strength of a trend. It’s particularly powerful for trading bots as it helps identify the type of market being experiences — and therefore which algorithm should be used.
For instance, when the ADX is low, this can indicate that market is trading in a range (known as trading sideways). This can indicate to your trading bot that it should NOT select a momentum-based trading algorithm. Alternatively, when the ADX is above 50, it can indicate that the market is gaining momentum…indicating a great time to switch to a momentum -based trading algorithm.
Adding this technical indicator to your trading bot can be a great way to help you automate more of your trading decision making.
You can read more about it here.
In this article, I’m going to show you how to add the ADX indicator to your trading bot.
In this article, I’m going to show you how to add the ADX to your auto trading bot. I’ll be demonstrating it on US Market data from Alpaca Markets, although you can also use it on any other candlestick data.
P.S. If you’ve added other indicators from my previous articles or YouTube videos, skip this step.
Technical indicators seem to be an ever-growing science. TA Lib alone has over 100 different technical indicators, and there are plenty of custom ones available.
Therefore, our trading bot must be able to handle multiple technical indicators
We’ll start by adding a file called indicators.py
to our trading bot. This file will handle all of our indicators.
Add the file by navigating to your trading bot dev environment and adding a file called indicators.py
Python Pandas makes data analysis exceptionally simple. Given that a trading bot is ALL about the efficient and effective analysis of data, I can’t think of a better library to be adding to your trading bot. You’ll use it over and over again if you follow my content!
Adding it is straightforward.
requirements.txt
pandas
to the bottompip install -r requirements.txt
in your terminalWithin the indicators.py
file you added earlier, we’re going to add some indicator routing. We will take care of adding the actual indicator a bit later.
This indicator routing is responsible for the following:
indicator_name
stringNote. If you want to see what this indicator file looks like when it has tons of indicators, check out this file on the TradeOxy Trading Bot. It’s the same repo I reference in all my content.
Here’s the code to add this routing:
Note the use of the **kwargs
argument in the function. It’s a super powerful little Python feature 🚀
Let’s define the ADX technical indicator function.
P.S. If you want to read more about the ADX technical indicator and how it can indicate market direction, check out this article from Investopedia.
Here’s the code:
Update your app.py
file in your trading bot to the following:
To see what the ADX technical indicator looks like when it runs, here’s a quick YouTube short from my tradeoxy-trading-bot open source repo:
The true power of trading bots is to hyperscale your analysis. For instance, even though you’ve built this little indicator in the last 10 minutes, you could EASILY do the following:
symbols
variable to be a listtimeframe
lineAll this and more is at your fingertips with a simple change of a line of code.