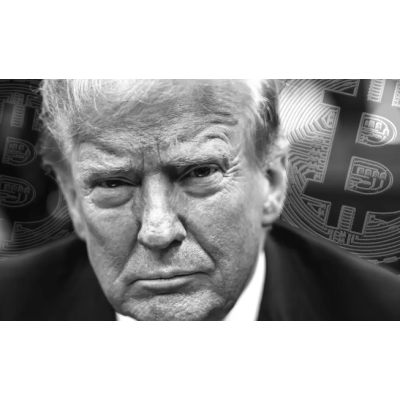
The Bollinger Bands technical indicator is a technical indicator that is widely used to calculate the movements of financial assets. Adding it to your trading bot can be a powerful way to analyze crypto, forex, and stock data patterns and use the insights you gain to level up your trading strategies.
In this episode, I’ll show you how to add the Bollinger Bands technical indicator to your trading bot and customize it for your trading bot strategies.
Algorithmic trading relies on analysis to inform decision-making. Almost always, this means adding some kind of indicator.
The thing about indicators and trading bots is that they tend to breed. The more you analyze data, the more likely it is that you’ll have more and more indicators you want to add to your trading bot.
A common way to manage this complexity is by building your own indicator library
In this library, you can add any indicator you choose.
It’s a powerful way to simplify much of your future autotrading development 🚀🚀🚀
If you haven’t done this in a previous episode, add the indicators.py
file to your trading bot. This file will handle anything to do with indicators in the rest of your TradeOxy Trading Bot development.
The Python Pandas Library is considered a gold standard in data analysis. It’s transcended the into the ranks of mythical status in Python and is widely used in an incredible set of industries.
Adding this exceptionally powerful analysis tool to your trading is as simple as 4 steps:
requirements.txt
pandas
to the bottompip install -r requirements.txt
Here’s what your should look like if you used my dev environment episode to get started:
To prepare for a multi-indicator future for your trading bot, we’re going to add some routing to your indicators.py
file. This routing allows you to simply specify the indicator you want to use at any stage of your algorithm by using normal human language.
Add this code to your indicators.py
(note that we’ll be adding in the Bollinger Bands technical indicator in the next section):
Here you can see:
**kwargs
special Python argument to allow us to add the components specific to each indicatorTo see how this might work when you have multiple indicators, check out the indicators.py
file in the main trading bot repo.
Now that we can route to the Bollinger Bands indicator, we can go ahead and add the code to calculate it.
I used the following default values for the Bollinger Bands indicator:
You could easily expand the standard deviation to provide a differential between the upper and lower standard deviations, but I’ve left that aside for a more advanced approach in a future episode.
P.S. A great reference article to read more about Bollinger Bands is from Investopedia here.
TA Lib is considered by many traders to be the go-to library for calculating technical indicators. It’s also a part of the dev environment.
The code below adds the Bollinger Bands indicator to your library using Python and TA Lib:
You’re all set!