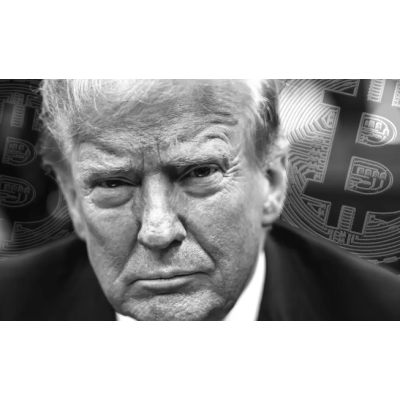
Creating a crypto trading bot can open up new ways of understanding the financial market dynamics and reap profits from automated trades. Today, we’re going to leverage the power of the Skale blockchain and Ruby Exchange, to create our own crypto trading bot.
Skale is a high-performance, Ethereum-compatible network that allows developers to run smart contracts at a fraction of the cost, with sub-second block times. Ruby Exchange, on the other hand, is a popular cryptocurrency exchange that provides a Ruby client for its API. By integrating these two technologies, we can create a robust, scalable, and efficient crypto trading bot.
Our ultimate goal is to develop a bot that can monitor price fluctuations in real time and execute trades according to a pre-set strategy. Along the way, we’ll gain insights into blockchain technology, cryptocurrency trading, and the essentials of automated trading systems.
Before diving into the coding aspect, it’s crucial to prepare your development environment. Install Ruby, Skale, and the necessary libraries on your system. With Ruby, it’s recommended to use a version manager like RVM or rbenv to manage different Ruby versions.
# Using RVM
\curl -sSL https://get.rvm.io | bash -s stable --ruby
# Using rbenv
brew install rbenv ruby-build
echo 'if which rbenv > /dev/null; then eval "$(rbenv init -)"; fi' >> ~/.bash_profile
source ~/.bash_profile
rbenv install 2.7.1
rbenv global 2.7.1
To set up the Skale network, visit their official documentation and follow the guidelines.
First, we authenticate with the Ruby Exchange API, which involves passing the API key and secret:
require 'ruby_exchange'
client = RubyExchange::Client.new(
api_key: 'your_api_key',
secret: 'your_secret',
)
# Confirm the client is working
puts client.ping # Should return {}
Next, we connect our bot to the Skale blockchain using the skale.rb library. We’ll use the private key of our account to do so.
require 'skale'
skale = Skale::RPC.new('http://your_skale_endpoint', 'your_private_key')
The core of our bot is the trading logic. We’ll build a simple strategy where our bot buys a specific amount of a coin when its price drops by a certain percentage and sells when the price increases by a certain percentage.
def trade_logic(client, coin, buy_threshold, sell_threshold, quantity)
coin_info = client.get_ticker(symbol: "#{coin}USDT")
last_price = coin_info.last_price
if coin_info.price_change_percent <= -buy_threshold
client.create_order(
symbol: "#{coin}USDT",
side: 'BUY',
type: 'MARKET',
quantity: quantity
)
elsif coin_info.price_change_percent >= sell_threshold
client.create_order(
symbol: "#{coin}USDT",
side: 'SELL',
type: 'MARKET',
quantity: quantity
)
end
end
We’ll use the ‘rufus-scheduler’ gem to run our trading logic every minute:
require 'rufus-scheduler'
scheduler = Rufus::Scheduler.new
scheduler.every '1m' do
trade_logic(client, 'BTC', -3, 3, 0.001)
end
scheduler.join
In a real-world scenario, things can go wrong. You may lose internet connectivity, the exchange may become unreachable, or a trade may fail. It’s essential to handle these errors gracefully and not allow them to crash your bot.
begin
scheduler.every '1m' do
trade_logic(client, 'BTC', -3, 3, 0.001)
end
rescue StandardError => e
puts "An error occurred: #{e.message}"
# Reattempt connection or notify the user about the error.
end
Trading limits are another essential feature. They prevent your bot from placing trades that could empty your account if something goes wrong with your trading strategy.
def trade_logic(client, coin, buy_threshold, sell_threshold, quantity)
# ...
account_info = client.get_account_info
# Check balance before buying
if coin_info.price_change_percent <= -buy_threshold && account_info['free'] >= quantity
# Place buy order
# ...
# Check balance before selling
elsif coin_info.price_change_percent >= sell_threshold && account_info['free'] >= quantity
# Place sell order
end
end
Adding logging to your bot is a simple way to track its performance and identify issues. For Ruby, there’s a built-in ‘logger’ library that’s easy to use.
require 'logger'
logger = Logger.new('bot.log')
begin
# Trading logic
rescue StandardError => e
logger.error("An error occurred: #{e.message}")
end
While running your bot on your local machine is okay for testing, you’ll want to deploy it to a server for long-term use. There are numerous options for this, such as AWS, Google Cloud, and Heroku. Some hosting services even offer specific features for bots.
Before deploying your bot, it’s crucial to thoroughly test it. Many exchanges offer ‘paper trading’, where you can test your bot using simulated money. This allows you to refine your strategy and identify bugs without risking real money.
The trading logic in our basic bot was quite simple, based on fixed thresholds. In reality, most successful trading bots use much more complex strategies. These might involve multiple technical indicators, statistical analysis, or even machine learning models.
For instance, instead of simply buying when the price drops a certain amount, you might look for ‘buy signals’ from several different indicators. This could include moving averages, relative strength index (RSI), Bollinger bands, or many others.
In our example, we used ‘market’ orders, which are fulfilled immediately at the current market price. However, depending on your trading strategy, you might want to use ‘limit’ orders. These orders are only executed when the price reaches the level you specify, which can be useful in certain strategies.
Before deploying a new strategy, you’ll want to test it on historical data to see how it would have performed. This process is known as ‘backtesting’. It’s not a perfect predictor of future performance, but it can help identify strategies that are likely to be profitable.
A crucial part of any trading strategy is knowing when to exit a position. ‘Stop-loss’ and ‘take-profit’ orders are tools you can use to automatically close a position if the price hits a certain level, protecting your profits or limiting your losses.
While the REST API we used in our bot is sufficient for many purposes, you might need real-time data for certain strategies. Many exchanges provide WebSocket APIs that push real-time market data to your bot.
require 'ruby_exchange'
require 'rufus-scheduler'
require 'logger'
class TradingBot
attr_reader :client, :scheduler, :logger, :sma_window
def initialize(api_key, secret, sma_window = 14)
@client = RubyExchange::Client.new(api_key: api_key, secret: secret)
@scheduler = Rufus::Scheduler.new
@logger = Logger.new('bot.log')
@sma_window = sma_window
end
def run
begin
scheduler.every '1m' do
trade_logic('BTC', 0.001)
end
rescue StandardError => e
logger.error("An error occurred: #{e.message}")
# Handle error or retry
end
end
private
def trade_logic(coin, quantity)
coin_info = client.get_ticker(symbol: "#{coin}USDT")
account_info = client.get_account(symbol: "#{coin}USDT")
sma = calculate_sma(coin)
if coin_info.last_price < sma && account_info['free'] >= quantity
place_limit_order(coin, 'BUY', quantity, coin_info.last_price)
elsif coin_info.last_price > sma && account_info['free'] >= quantity
place_limit_order(coin, 'SELL', quantity, coin_info.last_price)
end
end
def calculate_sma(coin)
historical_prices = client.get_historical_klines(symbol: "#{coin}USDT", interval: '1m', limit: sma_window)
closing_prices = historical_prices.map { |kline| kline.close_price.to_f }
sma = closing_prices.sum / closing_prices.length
return sma
end
def place_limit_order(coin, side, quantity, price)
client.create_order(
symbol: "#{coin}USDT",
side: side,
type: 'LIMIT',
quantity: quantity,
price: price
)
end
end
# Run the bot
bot = TradingBot.new('your_api_key', 'your_secret')
bot.run
Building a crypto trading bot involves much more than just writing code. It’s a deep dive into financial markets, trading strategies, and the technology that enables it all. And while the road can be challenging, it can also be incredibly rewarding.
In this guide, we’ve given you a foundation to build upon, but it’s only the beginning. As with any software project, there’s always room for improvement, optimization, and new features. The world of cryptocurrency and blockchain is evolving at a breakneck pace, and the possibilities are virtually endless.
So don’t stop here. Keep learning, keep experimenting, and keep improving your bot. Whether you’re doing it for profit, for fun, or just to learn, building a crypto trading bot is a journey worth embarking on. Happy coding!