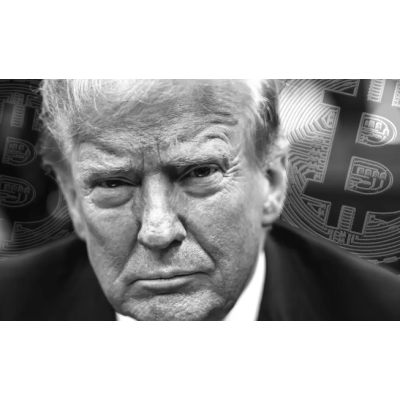
As we journey through the digital age, algorithms have taken a front-row seat in decision-making processes, even in financial trading. In the world of finance, algorithmic or algo-trading utilizes complex formulas, mathematical models, and human oversight to make high-speed, high-frequency decisions. Trading bots are one such implementation of algo-trading. This article will guide you on how to create a simple yet effective trading bot.
A trading bot is a software program that interacts with financial exchanges (often using APIs to obtain and interpret relevant information) and places buy or sell orders on your behalf based on the market data’s interpretation. These bots are designed to make trading decisions faster, eliminating emotional human factors, ensuring efficiency and accuracy.
Python is a language of choice due to its ease of use and the vast number of financial and scientific libraries available. We will be using the ccxt
library, which provides a unified way to interact with over 100 cryptocurrency exchanges. Install the necessary dependencies using pip:
pip install ccxt pandas
Before jumping into the code, it is important to define what your trading strategy will be. For our bot, we will be using a simple yet effective strategy called “moving average crossover”. This strategy dictates buying when the short-term moving average crosses above the long-term moving average, and selling when the reverse happens.
Using ccxt
, we can easily connect to the exchange. For this example, we'll be using Binance:
import ccxt
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
Replace 'YOUR_API_KEY'
and 'YOUR_SECRET_KEY'
with your actual API keys from Binance.
Our bot will make decisions based on the cryptocurrency’s historical price data. We’ll fetch this data from the Binance exchange using the fetch_ohlcv
method, which retrieves OHLCV (Open, High, Low, Close, Volume) data:
import pandas as pd
def fetch_data(symbol):
data = exchange.fetch_ohlcv(symbol, '1d') # 1 day data
header = ['Timestamp', 'Open', 'High', 'Low', 'Close', 'Volume']
df = pd.DataFrame(data, columns=header)
return df
To implement the moving average crossover strategy, we need to calculate the short-term and long-term moving averages:
def calculate_MA(df, short_term=50, long_term=200):
df['ShortTerm_MA'] = df['Close'].rolling(window=short_term).mean()
df['LongTerm_MA'] = df['Close'].rolling(window=long_term).mean()
return df
This code snippet calculates the short-term (50-day) and long-term (200-day) moving averages based on the closing prices. It then adds the moving averages to our data frame.
Next, we’ll define the function for our trading strategy:
def strategy(df):
buy_signals = []
sell_signals = []
for i in range(len(df)):
if df['ShortTerm_MA'].iloc[i] > df['LongTerm_MA'].iloc[i]:
buy_signals.append(df['Close'].iloc[i])
sell_signals.append(float('nan'))
elif df['ShortTerm_MA'].iloc[i] < df['LongTerm_MA'].iloc[i]:
sell_signals.append(df['Close'].iloc[i])
buy_signals.append(float('nan'))
else:
buy_signals.append(float('nan'))
sell_signals.append(float('nan'))
return buy_signals, sell_signals
This function iterates over the data frame, checking if the short-term MA is greater than the long-term MA (a signal to buy) or if the short-term MA is less than the long-term MA (a signal to sell).
Once we have our trading signals, we can use these signals to place orders on the exchange:
def place_order(symbol, signal):
if signal == 'buy':
exchange.create_market_buy_order(symbol, 1)
elif signal == 'sell':
exchange.create_market_sell_order(symbol, 1)
This function places a market buy or sell order on the exchange. Please note the quantity here is set to 1 as an example. It should be adjusted based on your specific trading parameters.
Now that we have defined our functions, we can bring it all together:
def main():
symbol = 'BTC/USDT'
df = fetch_data(symbol)
df = calculate_MA(df)
buy_signals, sell_signals = strategy(df)
for i in range(len(df)):
if buy_signals[i] != 'nan':
place_order(symbol, 'buy')
elif sell_signals[i] != 'nan':
place_order(symbol, 'sell')
if __name__ == "__main__":
main()
This main
function fetches the data, calculates the moving averages, gets the trading signals, and places the orders based on these signals.
Backtesting is a critical step in creating your trading bot. It involves testing your trading strategy against historical data to see how it would have performed. This helps in understanding the effectiveness of your trading strategy before deploying it in the real world.
def backtest(df, buy_signals, sell_signals):
balance = 100.0 # initial balance in USD
btc = 0 # BTC balance
for i in range(len(df)):
if buy_signals[i] is not None and balance > 0:
btc = balance / df['Close'].iloc[i] # Buy with all balance
balance = 0
elif sell_signals[i] is not None and btc > 0:
balance = btc * df['Close'].iloc[i] # Sell all BTC
btc = 0
return balance, btc
The results of backtesting can give you valuable insights into how your bot will perform. Based on these results, you may need to adjust your trading strategy. This could be changing your moving average periods, incorporating other indicators, or even developing a completely new strategy.
Robust error handling can save your bot from catastrophic failures. It’s also important to log the operations of your bot. Python’s built-in logging library makes it easy to add logging to your bot:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger()
def strategy(df):
# previous code...
except Exception as e:
logger.error(f"An error occurred: {str(e)}")
This setup allows you to catch and log any unexpected errors that may occur during the operation of your bot.
Up until now, we’ve been using historical data for backtesting. When you’re ready to start live trading, you’ll need to modify your bot to work with real-time data. One way to do this is by using WebSocket APIs provided by many cryptocurrency exchanges, which allow you to get real-time market data.
import asyncio
from ccxt.async_support.binance import binance
async def main():
exchange = binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
async with exchange.watch_trades('BTC/USDT') as trades:
while True:
trade = await trades.get()
print(trade)
if __name__ == "__main__":
asyncio.run(main())
This will print out the real-time trades happening in the BTC/USDT market.
Once your bot is thoroughly tested and ready to go live, you might want to consider deploying it to a cloud-based server. This way, it can run 24/7 without being dependent on your local machine.