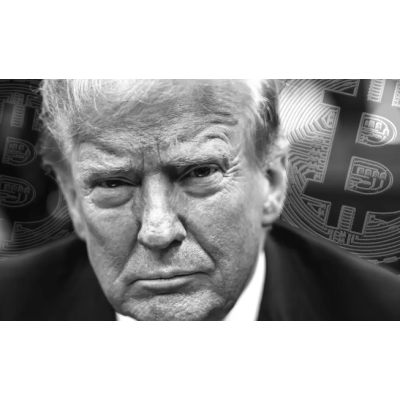
Commodity trading has always been a lucrative business, but it can also be quite challenging. With so many factors affecting prices, traders need to keep a constant eye on the market, identify patterns, and make quick decisions. However, with the help of technology, traders can now automate commodity trading using Python.
Python is a popular programming language in the world of finance and trading. It is easy to learn, versatile, and has a vast library of powerful tools for data analysis and visualization. In this article, we’ll show you how to build your own trading bot using Python.
Step 1: Setting Up Your Environment
To get started, you’ll need to set up your Python environment. We recommend using Anaconda, a popular distribution that comes with many of the necessary packages pre-installed. You can download Anaconda from the official website and follow the installation instructions.
Step 2: Collecting Data
The first step in building your trading bot is to collect data. You can use various sources to obtain the necessary data, such as Yahoo Finance, Quandl, or Alpha Vantage. In this example, we’ll use Alpha Vantage to obtain historical data for gold prices.
To use Alpha Vantage, you’ll need to sign up for a free API key. Once you have the key, you can use the following code to collect the data:
import pandas as pd
import requests
url = 'https://www.alphavantage.co/query?function=TIME_SERIES_DAILY_ADJUSTED&symbol=XAU&apikey=YOUR_API_KEY'
response = requests.get(url)
data = response.json()['Time Series (Daily)']
df = pd.DataFrame(data).transpose()
df.columns = ['open', 'high', 'low', 'close', 'adjusted_close', 'volume', 'dividend_amount', 'split_coefficient']
df.index = pd.to_datetime(df.index)
df = df.astype(float)
This code collects daily historical data for gold prices and converts it into a pandas DataFrame.
Step 3: Creating Trading Strategies
The next step is to create trading strategies. Trading strategies are sets of rules that dictate when to buy or sell a commodity. In this example, we’ll create a simple trading strategy based on the moving average crossover.
A moving average crossover is a popular technical analysis tool that uses two moving averages to identify trend changes. When the short-term moving average crosses above the long-term moving average, it is a signal to buy, and when it crosses below, it is a signal to sell.
We’ll use a 50-day moving average and a 200-day moving average to create our trading strategy. We’ll buy when the 50-day moving average crosses above the 200-day moving average and sell when the 50-day moving average crosses below the 200-day moving average.
df['50-day'] = df['adjusted_close'].rolling(window=50).mean()
df['200-day'] = df['adjusted_close'].rolling(window=200).mean()
df['signal'] = 0.0
df['signal'] = np.where(df['50-day'] > df['200-day'], 1.0, 0.0)
df['position'] = df['signal'].diff()
This code calculates the 50-day and 200-day moving averages and creates a signal column that is set to 1.0 when the 50-day moving average is above the 200-day moving average and 0.0 otherwise. It then creates a position column that takes the difference between the signal column and the previous signal column. This column represents the position of our trading bot, where 1.0 means it is long (i.e., buying), -1.0 means it is short (i.e., selling), and 0.0 means it is not holding any positions. With these columns, you can easily create trading strategies and test them using backtesting libraries like backtrader. Python’s flexibility and powerful data analysis capabilities make it an excellent tool for automating commodity trading and other financial applications.
Step 4: Backtesting
Once you have your trading strategy, you’ll need to test it to see how it performs. Backtesting is the process of applying your trading strategy to historical data to see how it would have performed.
In Python, you can use the backtrader library to perform backtesting. Backtrader is a powerful backtesting framework that allows you to test your trading strategies against historical data.
import backtrader as bt
class SimpleMovingAverageCross(bt.Strategy):
params = (('sma1', 50), ('sma2', 200))
def __init__(self):
self.sma1 = bt.indicators.SimpleMovingAverage(self.data.close, period=self.params.sma1)
self.sma2 = bt.indicators.SimpleMovingAverage(self.data.close, period=self.params.sma2)
self.crossover = bt.indicators.CrossOver(self.sma1, self.sma2)
def next(self):
if not self.position:
if self.crossover > 0:
self.buy()
elif self.crossover < 0:
self.close()
cerebro = bt.Cerebro()
data = bt.feeds.PandasData(dataname=df)
cerebro.adddata(data)
cerebro.addstrategy(SimpleMovingAverageCross)
cerebro.run()
This code defines a SimpleMovingAverageCross strategy class that uses the 50-day and 200-day moving averages to create buy and sell signals. It then uses the backtrader library to backtest the strategy against the historical data.
Step 5: Deploying Your Trading Bot
Once you have tested your trading bot and are satisfied with its performance, you can deploy it to start trading. There are various ways to deploy your trading bot, such as using a cloud-based platform like Amazon Web Services or running it on a local machine.
In this article, we showed you how to automate commodity trading using Python. We walked you through the steps of collecting data, creating trading strategies, backtesting, and deploying your trading bot. With the help of Python, you can now build your own trading bot and start trading commodities with confidence. Happy trading!