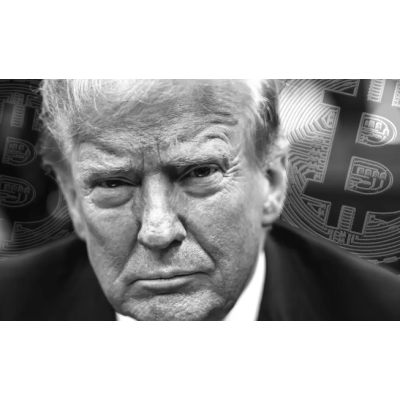
In this article, we will explore how to create a trading bot for Binance using Python and the Binance API. We will provide a step-by-step guide for implementing a simple yet effective trading strategy, as well as show how to backtest and evaluate the strategy’s performance using historical data. Finally, we will demonstrate how to implement a live trading mode, allowing the bot to execute trades in real-time. By following the instructions provided in this article, you can create a powerful tool that will automate your trading and potentially increase your profits.
The first step is to create a Binance account. If you don’t have an account yet, you can use my referral link to create a Binance account so we can both earn up to 100 USDT trading fee credit.
Once you have created an account, go to the API Management page and create a new API key. Make sure to keep the API key and secret key safe, as they will be used to access your Binance account from the Python script.
To build the Binance crypto bot, you will need to install some Python libraries. Open a command prompt or terminal and run the following command to install the required libraries:
pip install pandas numpy matplotlib python-binance
After installing the required libraries, import them in the Python script as follows:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from binance.client import Client
To connect to the Binance API, create a new instance of the Client class and pass your API key and secret key as input parameters:
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_SECRET_KEY'
client = Client(api_key, api_secret)
The strategy is the heart of the crypto bot. In this example, we will use a simple strategy that uses two technical indicators to generate buy and sell signals. The two indicators are the Simple Moving Average (SMA) and the Relative Strength Index (RSI).
# Example strategy
def strategy(df):
buy = df['SMA'] > df['SMA'].shift(1)
buy &= df['RSI'] < 30
sell = df['SMA'] < df['SMA'].shift(1)
sell |= df['RSI'] > 70
return buy, sell
We will generate the buy signal when the current SMA is higher than the previous SMA, and the RSI is below 30. The sell signal is generated when the current SMA is lower than the previous SMA or the RSI is above 70.
To calculate the technical indicators, we need to download the historical price data from the Binance API and apply the required calculations. Here, we will calculate the SMA and RSI.
# Calculate Technical Indicators
def calculate_indicators(df):
# Simple Moving Average (SMA)
sma = df['close'].rolling(window=50).mean()
df['SMA'] = sma
# Relative Strength Index (RSI)
delta = df['close'].diff()
gain = delta.where(delta > 0, 0)
loss = -delta.where(delta < 0, 0)
avg_gain = gain.rolling(window=14).mean()
avg_loss = loss.rolling(window=14).mean()
rs = avg_gain / avg_loss
rsi = 100 - (100 / (1 + rs))
df['RSI'] = rsi
Here, we first calculate the SMA using a 50-day window by taking the rolling mean of the close price. We then calculate the RSI by first calculating the price changes (delta), and then calculating the average gain and loss over a 14-day window. We then calculate the relative strength (rs) as the ratio of the average gain to the average loss, and use this value to calculate the RSI.
To download the historical price data from the Binance API, we can use the following code:
# Download Binance historical data
def download_data(symbol, interval, start_str):
print(f'Downloading data for {symbol}. Interval {interval}. Starting from {start_str}')
klines = client.get_historical_klines(symbol, interval, start_str)
data = pd.DataFrame(klines, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume', 'close_time', 'quote_asset_volume', 'number_of_trades', 'taker_buy_base_asset_volume', 'taker_buy_quote_asset_volume', 'ignore'])
data['timestamp'] = pd.to_datetime(data['timestamp'], unit='ms')
data.set_index('timestamp', inplace=True)
data['close'] = data['close'].astype(float)
return data
Here, we use the client.get_historical_klines
function to download the historical price data for the given symbol and interval. We then convert the data to a pandas DataFrame and set the timestamp column as the index. Finally, we convert the close
column to a float data type.
Once we have downloaded the historical price data and calculated the technical indicators, we can backtest the strategy by generating the buy and sell signals based on the strategy function, and simulating trades based on these signals.
# Backtesting strategy
def backtest(data):
calculate_indicators(data)
buy, sell = strategy(data)
data['buy'] = buy
data['sell'] = sell
data['position'] = np.nan
data.loc[buy, 'position'] = 1
data.loc[sell, 'position'] = 0
data['position'].fillna(method='ffill', inplace=True)
data['position'].fillna(0, inplace=True)
data['returns'] = np.log(data['close'] / data['close'].shift(1))
data['strategy'] = data['position'].shift(1) * data['returns']
data['cumulative_returns'] = data['strategy'].cumsum().apply(np.exp)
return data
Here, we first call the calculate_indicators
function to calculate the technical indicators. We then call the strategy
function to generate the buy and sell signals. We create a new column called position
to represent our trading position, and set the values to 1 for buy signals, 0 for sell signals, and NaN for no signals. We then fill the NaN values using forward filling (ffill
) to simulate holding the position until the next signal, and fill remaining NaN values with 0 to simulate closing the position. We calculate the daily log returns and multiply them by the trading position to simulate the strategy returns. We then calculate the cumulative returns by taking the exponential of the cumsum of the strategy returns.
Finally, we can plot the cumulative returns to visualize the performance of the strategy.
# Plot Cumulative Returns
def plot_results(data):
data[['cumulative_returns']].plot(figsize=(10, 6))
plt.xlabel('Date')
plt.ylabel('Cumulative Returns')
plt.title('Backtesting Results')
plt.show()
Here, we simply plot the cumulative_returns
column of the data DataFrame.
To run the backtesting you simply need to call the following test_trading()
function:
# Run Test Trading mode
def test_trading():
data = download_data('BTCUSDT', '5m', '1 Jan 2022')
data = backtest(data)
plot_results(data)
return data
This function will download the historical data, run the backtest, and then plot the results. The backtest
function will simulate trading using the same strategy, but using the historical data to generate the buy and sell signals instead of real-time data. This allows you to evaluate the performance of your strategy using historical data.
Below are the results for the example BTC-USDT strategy using 3m data from 1 January 2022 to the date of this writing, 21 Feb 2023:
To run the crypto bot in live mode, you can use the following code:
Live Trading Method We will be using the Binance API to connect to the exchange, and the Python programming language to implement our trading strategy. Our trading strategy will involve the use of two technical indicators: the Exponential Moving Average (EMA) and the Relative Strength Index (RSI).
The following code will use the Binance API to perform live trading with our crypto bot:
# Live Trading mode
def live_trading():
client = Client(api_key, api_secret)
prev_buy_signal = False
prev_sell_signal = False
while True:
# Download data, calculate indicators and get signals
data = download_data('BTCUSDT', '5m', '1 Feb 2023')
calculate_indicators(data)
buy_signal, sell_signal = strategy(data)
buy_signal = buy_signal[-1]
sell_signal = sell_signal[-1]
if buy_signal and not prev_buy_signal:
order = client.create_test_order(
symbol='BTCUSDT',
side='BUY',
type='MARKET',
quantity=0.001,
)
print('Buy signal generated. Placing market buy order.')
elif sell_signal and not prev_sell_signal:
order = client.create_test_order(
symbol='BTCUSDT',
side='SELL',
type='MARKET',
quantity=0.001,
)
print('Sell signal generated. Placing market sell order.')
prev_buy_signal = buy_signal
prev_sell_signal = sell_signal
time.sleep(60)
In the live_trading
function, we first initialize the Binance API client with our api_key
and api_secret
. We then set prev_buy_signal
and prev_sell_signal
to False
to indicate that we have not generated any signals yet.
Inside the while
loop, we download the most recent data using the download_data
function with a time interval of 5 minutes starting from February 1, 2023. We then calculate the EMA and RSI using the calculate_indicators
function and generate the buy and sell signals using the strategy
function. We use only the latest signal using [-1]
.
If a buy signal is generated and we did not have a previous buy signal, the bot will create a test order to buy 0.001 BTC at the current market price. Similarly, if a sell signal is generated and we did not have a previous sell signal, the bot will create a test order to sell 0.001 BTC at the current market price. We use the create_test_order
function to create a test order to prevent actual trades from being executed. We also print a message indicating whether a buy or sell signal has been generated and that we are placing a market order.
Finally, we update prev_buy_signal
and prev_sell_signal
to reflect the current signals and wait for 60 seconds before repeating the process.
In this article, we have shown how to create a trading bot for Binance using Python and the Binance API. We have presented a simple yet effective trading strategy that uses two technical indicators to generate buy and sell signals for BTC/USDT. The strategy was implemented using Python, and we have provided step-by-step instructions for how to download the data, calculate the indicators, generate the signals, and execute trades using the Binance API.
We have also demonstrated how to perform backtesting on the strategy using historical data, which is an essential step in evaluating the effectiveness of any trading strategy. By simulating trading using historical data, we can gain valuable insights into the performance of our strategy and make more informed trading decisions.
Finally, we have shown how to implement a live trading mode that allows the trading bot to execute trades in real-time based on the buy and sell signals generated by the strategy. We have also provided a testing mode that allows us to evaluate the performance of the strategy using historical data.
In conclusion, building a trading bot using Python and the Binance API is a powerful way to automate your trading and potentially increase your profits. However, it is important to remember that trading is always risky, and you should never risk more than you can afford to lose. By using the tools and strategies presented in this article, you can make more informed trading decisions and potentially increase your chances of success.