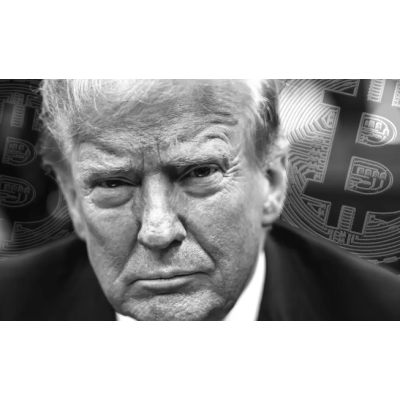
Welcome to my new series on automated trading using Coinbase and Python 3.
Automated trading can be a lot of fun. It combines quantitative analysis, scripting, and pattern identification disciplines to develop various strategies. When you find a solid strategy it can also be incredibly lucrative!
In this series, I show you how to build your own crypto trading bot to detect a market signal.
The series uses Python 3 to connect to Coinbase, then implements a modified version of the well-known Engulfing Candle Trading Strategy. Throughout the project, I show you how to set up your project in a way that makes it easy to expand with your own ideas and strategies in the future.
All code for the series can be found on the project GitHub. Each episode has working code samples you can copy and paste straight into your own crypto trading bot. I only ask that you throw me a shoutout and a follow on Medium, LinkedIn, or Twitter.
To help you check out your code, I’ve created several locations where the output from this tutorial is posted. The code checks the “BTC-USDC” trading pair and can be found in the #coinbase and #strategyone areas. Check it out on a website, Twitter, and Discord. Note. All trading is at your own risk. Do your own research.
By the end of this episode, you’ll have set up your project for the rest of the series. You’ll learn how to import your sensitive settings in a reasonably secure manner.
An effective code base is extensible, manageable, and easily upgradeable. This is particularly important for an algorithmic trading bot as it enables you to rapidly add new indicators, strategies, and quantitative analysis outcomes to your bot.
A common way to section a code base is through functional areas. This allows you to group similar types of code functionality together, making it easier for you to manage.
This is the approach we’ll be using for this series. To get started, create a series of folders in your project titled as follows:
By the way, if you’re looking for a great IDE, I use Pycharm. It’s an excellent IDE, has great code completion, and will help you keep your code nice and PEP8 compliant. The Community Edition is free if you’re not using it for commercial purposes.
Next, create a file called main.py
in your folder. In this file add the following:
Any live trading requires you to interface with an exchange. Even if it’s an Automatic Money Maker like PancakeSwap, you’ll still need to somehow interact with a live capital market.
Inevitably, these exchanges require a credential exchange. This allows the exchange to verify that you (or your trading bot) are who you say you are and are authorized to do what you want to do. Your trading bot will require these credentials, and we need to store them in a safe manner.
Safe storage of credentials can be done through several methods. You can use a credential storage system like Google Cloud Secret Manager or use environmental variables.
For our project, we want a way that is reasonably secure and easily extensible. To achieve this, we’ll set up a .json
file, then import the settings into our program at program startup. This method means that the only time our credentials are exposed is:
While not foolproof, this approach offers a reasonable balance between security and functionality. It is suitable for at-home personal use (although if you’re dealing with large amounts of money or commercial applications I’d recommend a different solution).
Let’s do this now:
settings.json
on disk somewheresettings.json
to your .gitignore
file (if using Git or some other VCS)main.py
and add a function to import the settings and a variable with the settings.json
file location. Here’s what main.py
should look like:It might not seem like much, but you’ve made a solid start on your project! Nice work.
In the next episode, I’ll show you how to connect to Coinbase and start retrieving market data. See you there.